mirror of
https://github.com/kunkunsh/kunkun-ext-speech-to-text.git
synced 2025-07-18 20:01:30 +00:00
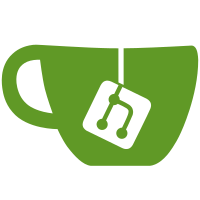
- Added project configuration files (tsconfig, eslint, vite, etc.) - Implemented Svelte5 frontend with routing - Created Deno backend for audio transcription using OpenAI Whisper - Added preferences page for API key configuration - Configured Tailwind CSS and theming - Implemented file selection and transcription functionality
24 lines
690 B
TypeScript
24 lines
690 B
TypeScript
import { expose } from '@kunkun/api/runtime/deno';
|
|
import OpenAI from '@openai/openai';
|
|
import type { API } from '../src/api.types';
|
|
|
|
expose({
|
|
transcribe: async (filepath: string, language: string) => {
|
|
const apiKey = Deno.env.get('OPENAI_API_KEY');
|
|
const openai = new OpenAI({ apiKey });
|
|
|
|
const fileData = await Deno.readFile(filepath);
|
|
|
|
// Convert to a File (filename is required)
|
|
const file = new File([fileData], 'audio.m4a', { type: 'audio/m4a' });
|
|
|
|
const transcription = await openai.audio.transcriptions.create({
|
|
file: file,
|
|
model: 'whisper-1',
|
|
language: language // this is optional but helps the model
|
|
});
|
|
|
|
return transcription.text;
|
|
}
|
|
} satisfies API);
|