mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-07-12 19:01:30 +00:00
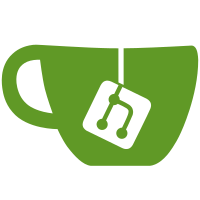
* Add loading animation to general settings * Update dependencies and integrate @tauri-store/svelte - Added `bon` and `bon-macros` packages to Cargo.lock. - Upgraded `tauri-plugin-svelte`, `tauri-store`, and related packages to their latest versions. - Updated `@tauri-store/svelte` integration in the desktop app, including changes to app configuration and layout handling. - Adjusted pnpm-lock.yaml to reflect updated package versions and added new dependencies. - Introduced a new app configuration file for development. * Enhance loading animation handling in FullScreenLoading component - Integrated conditional rendering for loading animations based on app configuration. - Updated default loading animation to "kunkun-dancing" in app configuration. - Adjusted general settings to ensure proper type handling for language labels. - Modified ui-iframe component to manage full-screen loading state more effectively. * remove a mis-placed config file * Refactor window handling to ensure focus after showing - Updated various components to use promise chaining with `show()` and `setFocus()` for better window management. - Introduced `data.win` in multiple places to streamline access to the current webview window. - Enhanced splashscreen and app layout handling to improve user experience by ensuring the window is focused after being shown. * Refactor window handling to improve safety and consistency - Introduced optional chaining for `data.win` to prevent potential runtime errors when accessing window methods. - Updated various components to ensure proper handling of window focus and visibility. - Enhanced the layout and extension pages to utilize the current webview window more effectively, improving overall user experience.
91 lines
2.5 KiB
Svelte
91 lines
2.5 KiB
Svelte
<script lang="ts">
|
|
import { Button } from "@kksh/svelte5"
|
|
import { Layouts } from "@kksh/ui"
|
|
import { LogicalSize } from "@tauri-apps/api/dpi"
|
|
import { getCurrentWebviewWindow } from "@tauri-apps/api/webviewWindow"
|
|
import { CircleX } from "lucide-svelte"
|
|
import { onMount } from "svelte"
|
|
import * as clipboard from "tauri-plugin-clipboard-api"
|
|
|
|
let image = $state<string | null>(null)
|
|
let { data } = $props()
|
|
let originalSize = $state<{ width: number; height: number } | null>(null)
|
|
let originalScaleFactor = $state<number | null>(null)
|
|
let scale = $state<number>(1)
|
|
let currentSize = $derived(
|
|
originalSize ? { width: originalSize.width * scale, height: originalSize.height * scale } : null
|
|
)
|
|
const win = getCurrentWebviewWindow()
|
|
|
|
$effect(() => {
|
|
if (currentSize) {
|
|
win.setSize(new LogicalSize(currentSize.width, currentSize.height))
|
|
}
|
|
})
|
|
|
|
async function getWindowSize() {
|
|
const size = await win.outerSize()
|
|
const scaleFactor = originalScaleFactor ?? (await win.scaleFactor())
|
|
const logicalSize = size.toLogical(scaleFactor)
|
|
return { logicalSize, scaleFactor }
|
|
}
|
|
|
|
onMount(async () => {
|
|
clipboard
|
|
.readImageBase64()
|
|
.then((b64) => {
|
|
image = b64
|
|
})
|
|
.finally(() => {
|
|
data.win?.show().then(() => data.win?.setFocus())
|
|
})
|
|
const { logicalSize, scaleFactor } = await getWindowSize()
|
|
originalSize = { width: logicalSize.width, height: logicalSize.height }
|
|
originalScaleFactor = scaleFactor
|
|
})
|
|
|
|
async function onWheel(e: WheelEvent) {
|
|
scale += (e.deltaY < 0 ? 1 : -1) * 0.05
|
|
}
|
|
|
|
function onGestureChange(e: Event) {
|
|
e.preventDefault()
|
|
// eslint-disable-next-line @typescript-eslint/no-explicit-any
|
|
scale = (e as any).scale
|
|
}
|
|
|
|
$effect(() => {
|
|
document.addEventListener("wheel", onWheel)
|
|
document.addEventListener("gesturechange", onGestureChange)
|
|
|
|
return () => {
|
|
document.removeEventListener("wheel", onWheel)
|
|
document.removeEventListener("gesturechange", onGestureChange)
|
|
}
|
|
})
|
|
</script>
|
|
|
|
<svelte:window
|
|
on:keydown={(e) => {
|
|
if (e.key === "Escape") {
|
|
win.close()
|
|
}
|
|
}}
|
|
/>
|
|
<Button size="icon" variant="ghost" class="fixed left-2 top-2" onclick={() => win.close()}>
|
|
<CircleX />
|
|
</Button>
|
|
<main class="z-50 h-screen w-screen overflow-hidden" data-tauri-drag-region>
|
|
{#if image}
|
|
<img
|
|
src={`data:image/png;base64,${image}`}
|
|
alt="screenshot"
|
|
class="pointer-events-none h-full w-full object-contain"
|
|
/>
|
|
{:else}
|
|
<Layouts.Center>
|
|
<p class="text-2xl font-bold">No image found in clipboard</p>
|
|
</Layouts.Center>
|
|
{/if}
|
|
</main>
|