mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-04-04 14:46:42 +00:00
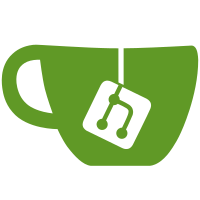
* feat: npm package registry API * refactor: move package registry files * refactor: move jsr and npm api to a new package * ci: add verify-package-export * test: implement tests for npm package validation as kunkun extension * chore: add missing dep for package-registry pkg * feat: make provenance an optional input for npm validation function * ci: add verify-package-export as dev dep to 2 packages that uses it * feat: add rekor log API, and return commit from jsr & npm package in validation function * feat: return github repo info from validation function of jsr and npm * feat: extend ExtPublishMetadata to include optional GitHub repository details * fix: eslint for ui package * refactor: format desktop * fix: eslint errors in desktop * format: all code * ci: add lint to CI * feat: add more info to validation function returned from package-registry npm jsr * pnpm lock * feat: add 2 more variables to supabase extension metadata model * format * feat: add provenance card * feat: add workflow path to ExtPublishMetadata and jsr/npm validation * update provenance * feat: make store extension and provenance more responsive * chore: add globals to ui package * fix: remove unnecessary any to fix eslint * fix: svg sanitize * chore: add @typescript-eslint/eslint-plugin to ui package to fix eslint * fix: update eslint dep to fix error * fix: try fixing eslint * fix: update eslint configuration for improved compatibility * chore: add globals package and update README for Discord invite * fix: update eslint rules and upgrade typescript-eslint dependency - Disabled additional eslint rules to resolve errors: - @typescript-eslint/no-unused-expressions - svelte/no-inner-declarations - Upgraded typescript-eslint from version 8.19.1 to 8.20.0 for improved compatibility. * update pnpm lock --------- Co-authored-by: Huakun Shen <huaukun.shen@huakunshen.com>
143 lines
3.3 KiB
TypeScript
143 lines
3.3 KiB
TypeScript
import * as v from "valibot"
|
|
|
|
export const NpmPkgVersionMetadata = v.object({
|
|
name: v.string(),
|
|
type: v.optional(v.string()),
|
|
license: v.optional(v.string()),
|
|
version: v.string(),
|
|
description: v.optional(v.string()),
|
|
dist: v.optional(
|
|
v.object({
|
|
integrity: v.string(),
|
|
shasum: v.optional(v.string()),
|
|
tarball: v.string(),
|
|
fileCount: v.optional(v.number()),
|
|
unpackedSize: v.optional(v.number()),
|
|
attestations: v.optional(
|
|
v.object({
|
|
url: v.string(),
|
|
provenance: v.object({
|
|
predicateType: v.string()
|
|
})
|
|
})
|
|
),
|
|
signatures: v.optional(
|
|
v.array(
|
|
v.object({
|
|
keyid: v.string(),
|
|
sig: v.string()
|
|
})
|
|
)
|
|
)
|
|
})
|
|
),
|
|
gitHead: v.optional(v.string()),
|
|
_npmUser: v.optional(
|
|
v.object({
|
|
name: v.string(),
|
|
email: v.string()
|
|
})
|
|
),
|
|
maintainers: v.optional(
|
|
v.array(
|
|
v.object({
|
|
name: v.string(),
|
|
email: v.string()
|
|
})
|
|
)
|
|
)
|
|
})
|
|
export type NpmPkgVersionMetadata = v.InferOutput<typeof NpmPkgVersionMetadata>
|
|
|
|
/**
|
|
* Full metadata of an npm package
|
|
* Sample URL: https://registry.npmjs.org/@huakunshen/jsr-client
|
|
*/
|
|
export const NpmPkgMetadata = v.object({
|
|
name: v.string(),
|
|
description: v.optional(v.string()),
|
|
"dist-tags": v.record(v.string(), v.string()), // latest, next, beta, rc
|
|
versions: v.record(v.string(), NpmPkgVersionMetadata),
|
|
time: v.objectWithRest(
|
|
{
|
|
created: v.string(),
|
|
modified: v.string()
|
|
},
|
|
v.string()
|
|
)
|
|
})
|
|
export type NpmPkgMetadata = v.InferOutput<typeof NpmPkgMetadata>
|
|
|
|
export const Provenance = v.object({
|
|
summary: v.object({
|
|
subjectAlternativeName: v.string(),
|
|
certificateIssuer: v.string(),
|
|
issuer: v.string(),
|
|
issuerDisplayName: v.string(),
|
|
buildTrigger: v.string(),
|
|
buildConfigUri: v.string(),
|
|
sourceRepositoryUri: v.string(),
|
|
sourceRepositoryDigest: v.string(),
|
|
sourceRepositoryRef: v.string(),
|
|
runInvocationUri: v.string(),
|
|
expiresAt: v.string(),
|
|
includedAt: v.string(),
|
|
resolvedSourceRepositoryCommitUri: v.string(),
|
|
transparencyLogUri: v.string(),
|
|
buildConfigDisplayName: v.string(),
|
|
resolvedBuildConfigUri: v.string(),
|
|
artifactName: v.string()
|
|
}),
|
|
sourceCommitResponseCode: v.number(),
|
|
sourceCommitUnreachable: v.boolean(),
|
|
sourceCommitNotFound: v.boolean()
|
|
})
|
|
export type Provenance = v.InferOutput<typeof Provenance>
|
|
|
|
export const NpmSearchResultObject = v.object({
|
|
downloads: v.object({
|
|
monthly: v.number(),
|
|
weekly: v.number()
|
|
}),
|
|
dependents: v.number(),
|
|
updated: v.string(),
|
|
searchScore: v.number(),
|
|
package: v.object({
|
|
name: v.string(),
|
|
keywords: v.array(v.string()),
|
|
version: v.string(),
|
|
description: v.optional(v.string()),
|
|
publisher: v.object({
|
|
email: v.string(),
|
|
username: v.string()
|
|
}),
|
|
maintainers: v.array(
|
|
v.object({
|
|
email: v.string(),
|
|
username: v.string()
|
|
})
|
|
),
|
|
license: v.optional(v.string()),
|
|
date: v.string(),
|
|
links: v.object({
|
|
npm: v.string()
|
|
})
|
|
}),
|
|
score: v.object({
|
|
final: v.number(),
|
|
detail: v.object({
|
|
popularity: v.number(),
|
|
quality: v.number(),
|
|
maintenance: v.number()
|
|
}),
|
|
flags: v.optional(
|
|
v.object({
|
|
insecure: v.number()
|
|
})
|
|
)
|
|
})
|
|
})
|
|
export type NpmSearchResultObject = v.InferOutput<typeof NpmSearchResultObject>
|
|
export const NpmSearchResults = v.object({ objects: v.array(NpmSearchResultObject) })
|
|
export type NpmSearchResults = v.InferOutput<typeof NpmSearchResults>
|