mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-04-04 14:46:42 +00:00
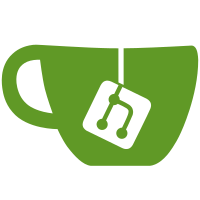
* feat: add page for add quick link (not working yet) * upgrade @kksh/svelte5 * fix: infinite recursive footer * dep: add @kksh/svelte5 to ui package * dep: add supabase-js * dep: add @iconify/svelte * style: modify StoreExtDetail width control * fixed: UI for extension store detail * feat: add page to create quick link * feat: display quick links in cmd palette * snapshot * show queries in command input * feat: quick link fully implemented * refactor: format all with prettier * feat: add icon picker for quick link adder * fix: make invert for icon optional, caused many types to crash
40 lines
1.1 KiB
TypeScript
40 lines
1.1 KiB
TypeScript
import { findAllArgsInLink } from "@/cmds/quick-links"
|
|
import { CmdTypeEnum } from "@kksh/api/models"
|
|
import type { AppState } from "@kksh/types"
|
|
import type { CmdValue } from "@kksh/ui/types"
|
|
import { derived, get, writable, type Writable } from "svelte/store"
|
|
|
|
export const defaultAppState: AppState = {
|
|
searchTerm: "",
|
|
highlightedCmd: ""
|
|
}
|
|
|
|
interface AppStateAPI {
|
|
clearSearchTerm: () => void
|
|
get: () => AppState
|
|
}
|
|
|
|
function createAppState(): Writable<AppState> & AppStateAPI {
|
|
const store = writable<AppState>(defaultAppState)
|
|
|
|
return {
|
|
...store,
|
|
get: () => get(store),
|
|
clearSearchTerm: () => {
|
|
store.update((state) => ({ ...state, searchTerm: "" }))
|
|
}
|
|
}
|
|
}
|
|
|
|
export const appState = createAppState()
|
|
|
|
// export const cmdQueries = derived(appState, ($appState) => {
|
|
// if ($appState.highlightedCmd.startsWith("{")) {
|
|
// const parsedCmd = JSON.parse($appState.highlightedCmd) as CmdValue
|
|
// if (parsedCmd.cmdType === CmdTypeEnum.QuickLink && parsedCmd.data) {
|
|
// return findAllArgsInLink(parsedCmd.data).map((arg) => ({ name: arg, value: "" }))
|
|
// }
|
|
// }
|
|
// return []
|
|
// })
|