mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-04-03 22:26:43 +00:00
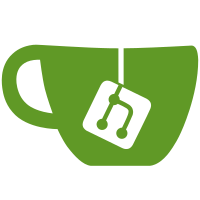
* feat: add drizzle orm * feat: update drizzle configuration and schema management - Added a check for DB_FILE_NAME in drizzle.config.ts to ensure it's set. - Updated package.json to change the package name to @kksh/drizzle and added exports for schema and relations. - Enhanced README.md with instructions for using the schema generation. - Refactored schema.ts for improved readability and organization of imports. * add tauri-plugin-sql * feat: add database select and execute commands - Introduced `select` and `execute` functions in the database module to facilitate querying and executing SQL commands. - Updated the Tauri plugin to expose these commands, allowing for database interactions from the frontend. - Added corresponding permissions for the new commands in the permissions configuration. - Enhanced the database library with JSON value handling for query parameters. * fix: sqlite select command * drizzle ORM verified working * refactor: clean up database module by removing unused SelectQueryResult type and disabling eslint for explicit any usage * pnpm lock update * Update enum definition for type safety - Changed enum to use 'as const' for better type inference - Ensured more robust handling of extension publish sources * reimplemented most db command functions with ORM (migrate from tauri command invoke * fixed searchExtensionData orm function * Refactor ORM commands and searchExtensionData function for improved readability and consistency - Reformatted import statements for better organization. - Cleaned up whitespace and indentation in searchExtensionData function. - Enhanced readability of SQL conditions and query building logic. - Disabled eslint for explicit any usage in the troubleshooters page. * Fix test assertions in database module to use array indexing for results format rust code * update deno lock * move drizzle from desktop to drizzle package * update pnpm version and lock * refactor: migrate db tauri commands to drizzle * refactor: remove unused extension and command CRUD operations from db module
183 lines
6.5 KiB
Rust
183 lines
6.5 KiB
Rust
use base64::prelude::*;
|
|
|
|
const COMMANDS: &[&str] = &[
|
|
"open_devtools",
|
|
"close_devtools",
|
|
"is_devtools_open",
|
|
"toggle_devtools",
|
|
"app_is_dev",
|
|
"open_trash",
|
|
"empty_trash",
|
|
"shutdown",
|
|
"reboot",
|
|
"sleep",
|
|
"toggle_system_appearance",
|
|
"show_desktop",
|
|
"quit_all_apps",
|
|
"sleep_displays",
|
|
"set_volume",
|
|
"turn_volume_up",
|
|
"turn_volume_down",
|
|
"toggle_stage_manager",
|
|
"toggle_bluetooth",
|
|
"toggle_hidden_files",
|
|
"eject_all_disks",
|
|
"logout_user",
|
|
"toggle_mute",
|
|
"mute",
|
|
"unmute",
|
|
"hide_all_apps_except_frontmost",
|
|
"get_selected_files_in_file_explorer",
|
|
"run_apple_script",
|
|
"run_powershell",
|
|
"get_applications",
|
|
"refresh_applications_list",
|
|
"refresh_applications_list_in_bg",
|
|
// "load_manifest",
|
|
// "load_all_extensions",
|
|
"path_exists",
|
|
"start_server",
|
|
"stop_server",
|
|
"restart_server",
|
|
"set_dev_extension_folder",
|
|
"set_extension_folder",
|
|
"get_extension_folder",
|
|
"get_dev_extension_folder",
|
|
"server_is_running",
|
|
/* -------------------------------------------------------------------------- */
|
|
/* FS */
|
|
/* -------------------------------------------------------------------------- */
|
|
"decompress_tarball",
|
|
"compress_tarball",
|
|
"unzip",
|
|
"copy_dir_all",
|
|
/* -------------------------------------------------------------------------- */
|
|
/* File Search */
|
|
/* -------------------------------------------------------------------------- */
|
|
"file_search",
|
|
/* -------------------------------------------------------------------------- */
|
|
/* Path */
|
|
/* -------------------------------------------------------------------------- */
|
|
"get_default_extensions_dir",
|
|
"get_default_extensions_storage_dir",
|
|
"is_window_label_registered",
|
|
"register_extension_window",
|
|
"register_extension_spawned_process",
|
|
"unregister_extension_spawned_process",
|
|
"unregister_extension_window",
|
|
"spawn_extension_file_server",
|
|
"get_ext_label_map",
|
|
// "ext_store_wrapper_set",
|
|
// "ext_store_wrapper_get",
|
|
// "ext_store_wrapper_has",
|
|
// "ext_store_wrapper_delete",
|
|
// "ext_store_wrapper_clear",
|
|
// "ext_store_wrapper_reset",
|
|
// "ext_store_wrapper_keys",
|
|
// "ext_store_wrapper_values",
|
|
// "ext_store_wrapper_entries",
|
|
// "ext_store_wrapper_length",
|
|
// "ext_store_wrapper_load",
|
|
// "ext_store_wrapper_save",
|
|
"get_server_port",
|
|
/* ----------------------------- sqlite database ---------------------------- */
|
|
// "create_extension",
|
|
// "get_all_extensions",
|
|
// "get_unique_extension_by_identifier",
|
|
// "get_unique_extension_by_path",
|
|
// "get_all_extensions_by_identifier",
|
|
// "delete_extension_by_path",
|
|
// "delete_extension_by_ext_id",
|
|
// "create_command",
|
|
// "get_command_by_id",
|
|
// "get_commands_by_ext_id",
|
|
// "delete_command_by_id",
|
|
// "update_command_by_id",
|
|
// "create_extension_data",
|
|
// "get_extension_data_by_id",
|
|
// "search_extension_data",
|
|
// "delete_extension_data_by_id",
|
|
// "update_extension_data_by_id",
|
|
"select",
|
|
"execute",
|
|
/* -------------------------------- Clipboard ------------------------------- */
|
|
"add_to_history",
|
|
"get_history",
|
|
/* -------------------------------------------------------------------------- */
|
|
/* Utils */
|
|
/* -------------------------------------------------------------------------- */
|
|
"plist_to_json",
|
|
/* -------------------------------------------------------------------------- */
|
|
/* Security */
|
|
/* -------------------------------------------------------------------------- */
|
|
"verify_auth",
|
|
"request_screen_capture_access",
|
|
"check_screen_capture_access",
|
|
/* -------------------------------------------------------------------------- */
|
|
/* MDNS */
|
|
/* -------------------------------------------------------------------------- */
|
|
"get_peers",
|
|
/* -------------------------------------------------------------------------- */
|
|
/* File Transfer */
|
|
/* -------------------------------------------------------------------------- */
|
|
"get_file_transfer_bucket_keys",
|
|
"get_file_transfer_bucket_by_key",
|
|
"local_net_send_file",
|
|
"download_files",
|
|
"file_transfer_preview_bucket",
|
|
/* -------------------------------------------------------------------------- */
|
|
/* Window */
|
|
/* -------------------------------------------------------------------------- */
|
|
"set_transparent_titlebar",
|
|
];
|
|
|
|
fn main() {
|
|
// SSL Cert
|
|
let cert_pem = match std::env::var("CERT_PEM") {
|
|
Ok(cert) => cert.into_bytes(),
|
|
Err(_) => include_bytes!("./self_signed_certs/cert.pem").to_vec(),
|
|
};
|
|
let key_pem = match std::env::var("KEY_PEM") {
|
|
Ok(key) => key.into_bytes(),
|
|
Err(_) => include_bytes!("./self_signed_certs/key.pem").to_vec(),
|
|
};
|
|
|
|
println!(
|
|
"cargo:rustc-env=BASE64_CERT_PEM={}",
|
|
BASE64_STANDARD.encode(cert_pem)
|
|
);
|
|
println!(
|
|
"cargo:rustc-env=BASE64_KEY_PEM={}",
|
|
BASE64_STANDARD.encode(key_pem)
|
|
);
|
|
|
|
// GRPC
|
|
let out_dir = std::path::PathBuf::from(std::env::var("OUT_DIR").unwrap());
|
|
tonic_build::configure()
|
|
.file_descriptor_set_path(out_dir.join("kk_grpc.bin"))
|
|
.compile(
|
|
&[
|
|
"../../grpc/protos/file-transfer.proto",
|
|
"../../grpc/protos/kunkun.proto",
|
|
],
|
|
&["../../grpc/protos"],
|
|
)
|
|
.expect("Failed to compile protos");
|
|
|
|
// Server Public Key
|
|
let raw_server_public_key = include_bytes!("./keys/server_public_key.pem").to_vec();
|
|
println!(
|
|
"cargo:rustc-env=BASE64_SERVER_PUBLIC_KEY={}",
|
|
BASE64_STANDARD.encode(raw_server_public_key)
|
|
);
|
|
println!(
|
|
"cargo:rustc-env=KUNKUN_PUBLISH={}",
|
|
std::env::var("KUNKUN_PUBLISH").unwrap_or("false".to_string())
|
|
);
|
|
|
|
tauri_plugin::Builder::new(COMMANDS)
|
|
.android_path("android")
|
|
.ios_path("ios")
|
|
.build();
|
|
}
|