mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-04-03 22:26:43 +00:00
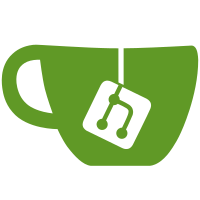
* feat: add drizzle orm * feat: update drizzle configuration and schema management - Added a check for DB_FILE_NAME in drizzle.config.ts to ensure it's set. - Updated package.json to change the package name to @kksh/drizzle and added exports for schema and relations. - Enhanced README.md with instructions for using the schema generation. - Refactored schema.ts for improved readability and organization of imports. * add tauri-plugin-sql * feat: add database select and execute commands - Introduced `select` and `execute` functions in the database module to facilitate querying and executing SQL commands. - Updated the Tauri plugin to expose these commands, allowing for database interactions from the frontend. - Added corresponding permissions for the new commands in the permissions configuration. - Enhanced the database library with JSON value handling for query parameters. * fix: sqlite select command * drizzle ORM verified working * refactor: clean up database module by removing unused SelectQueryResult type and disabling eslint for explicit any usage * pnpm lock update * Update enum definition for type safety - Changed enum to use 'as const' for better type inference - Ensured more robust handling of extension publish sources * reimplemented most db command functions with ORM (migrate from tauri command invoke * fixed searchExtensionData orm function * Refactor ORM commands and searchExtensionData function for improved readability and consistency - Reformatted import statements for better organization. - Cleaned up whitespace and indentation in searchExtensionData function. - Enhanced readability of SQL conditions and query building logic. - Disabled eslint for explicit any usage in the troubleshooters page. * Fix test assertions in database module to use array indexing for results format rust code * update deno lock * move drizzle from desktop to drizzle package * update pnpm version and lock * refactor: migrate db tauri commands to drizzle * refactor: remove unused extension and command CRUD operations from db module
56 lines
1.4 KiB
TypeScript
56 lines
1.4 KiB
TypeScript
/* eslint-disable @typescript-eslint/no-explicit-any */
|
|
import { sql } from "@kksh/api/commands"
|
|
import { error } from "@tauri-apps/plugin-log"
|
|
import { drizzle } from "drizzle-orm/sqlite-proxy"
|
|
import * as schema from "../drizzle/schema"
|
|
|
|
/**
|
|
* The drizzle database instance.
|
|
*/
|
|
export const db = drizzle<typeof schema>(
|
|
async (sqlQuery, params, method) => {
|
|
let rows: any = []
|
|
let results = []
|
|
console.log({
|
|
sql: sqlQuery,
|
|
params,
|
|
method
|
|
})
|
|
console.log(sqlQuery)
|
|
// If the query is a SELECT, use the select method
|
|
if (isSelectQuery(sqlQuery)) {
|
|
rows = await sql.select(sqlQuery, params).catch((e) => {
|
|
error("SQL Error:", e)
|
|
return []
|
|
})
|
|
} else {
|
|
// Otherwise, use the execute method
|
|
rows = await sql.execute(sqlQuery, params).catch((e) => {
|
|
error("SQL Error:", e)
|
|
return []
|
|
})
|
|
return { rows: [] }
|
|
}
|
|
|
|
rows = rows.map((row: any) => {
|
|
return Object.values(row)
|
|
})
|
|
|
|
// If the method is "all", return all rows
|
|
results = method === "all" ? rows : rows[0]
|
|
return { rows: results }
|
|
},
|
|
// Pass the schema to the drizzle instance
|
|
{ schema: schema, logger: true }
|
|
)
|
|
|
|
/**
|
|
* Checks if the given SQL query is a SELECT query.
|
|
* @param sql The SQL query to check.
|
|
* @returns True if the query is a SELECT query, false otherwise.
|
|
*/
|
|
function isSelectQuery(sql: string): boolean {
|
|
const selectRegex = /^\s*SELECT\b/i
|
|
return selectRegex.test(sql)
|
|
}
|