mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-04-03 22:26:43 +00:00
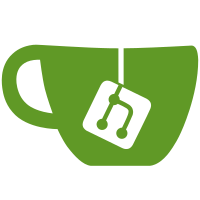
* feat: add drizzle orm * feat: update drizzle configuration and schema management - Added a check for DB_FILE_NAME in drizzle.config.ts to ensure it's set. - Updated package.json to change the package name to @kksh/drizzle and added exports for schema and relations. - Enhanced README.md with instructions for using the schema generation. - Refactored schema.ts for improved readability and organization of imports. * add tauri-plugin-sql * feat: add database select and execute commands - Introduced `select` and `execute` functions in the database module to facilitate querying and executing SQL commands. - Updated the Tauri plugin to expose these commands, allowing for database interactions from the frontend. - Added corresponding permissions for the new commands in the permissions configuration. - Enhanced the database library with JSON value handling for query parameters. * fix: sqlite select command * drizzle ORM verified working * refactor: clean up database module by removing unused SelectQueryResult type and disabling eslint for explicit any usage * pnpm lock update * Update enum definition for type safety - Changed enum to use 'as const' for better type inference - Ensured more robust handling of extension publish sources * reimplemented most db command functions with ORM (migrate from tauri command invoke * fixed searchExtensionData orm function * Refactor ORM commands and searchExtensionData function for improved readability and consistency - Reformatted import statements for better organization. - Cleaned up whitespace and indentation in searchExtensionData function. - Enhanced readability of SQL conditions and query building logic. - Disabled eslint for explicit any usage in the troubleshooters page. * Fix test assertions in database module to use array indexing for results format rust code * update deno lock * move drizzle from desktop to drizzle package * update pnpm version and lock * refactor: migrate db tauri commands to drizzle * refactor: remove unused extension and command CRUD operations from db module
89 lines
2.4 KiB
TypeScript
89 lines
2.4 KiB
TypeScript
import { sql } from "drizzle-orm"
|
|
import {
|
|
blob,
|
|
foreignKey,
|
|
integer,
|
|
numeric,
|
|
primaryKey,
|
|
sqliteTable,
|
|
text
|
|
} from "drizzle-orm/sqlite-core"
|
|
|
|
export const schemaVersion = sqliteTable("schema_version", {
|
|
version: integer().notNull()
|
|
})
|
|
|
|
export const extensions = sqliteTable("extensions", {
|
|
extId: integer("ext_id").primaryKey({ autoIncrement: true }),
|
|
identifier: text().notNull(),
|
|
version: text().notNull(),
|
|
// enabled: numeric().default(sql`(TRUE)`),
|
|
enabled: integer({ mode: "boolean" }),
|
|
path: text(),
|
|
data: numeric(),
|
|
installedAt: numeric("installed_at").default(sql`(CURRENT_TIMESTAMP)`)
|
|
})
|
|
|
|
export const commands = sqliteTable("commands", {
|
|
cmdId: integer("cmd_id").primaryKey({ autoIncrement: true }),
|
|
extId: integer("ext_id")
|
|
.notNull()
|
|
.references(() => extensions.extId, { onDelete: "cascade" }),
|
|
name: text().notNull(),
|
|
enabled: integer({ mode: "boolean" }),
|
|
// enabled: numeric().default(sql`(TRUE)`),
|
|
alias: text(),
|
|
hotkey: text(),
|
|
type: text().notNull(),
|
|
data: numeric()
|
|
})
|
|
|
|
export const extensionData = sqliteTable("extension_data", {
|
|
dataId: integer("data_id").primaryKey({ autoIncrement: true }),
|
|
extId: integer("ext_id")
|
|
.notNull()
|
|
.references(() => extensions.extId, { onDelete: "cascade" }),
|
|
dataType: text("data_type").notNull(),
|
|
// data: text({ mode: "json" }).notNull(),
|
|
// metadata: text({ mode: "json" }),
|
|
data: text("data").notNull(),
|
|
metadata: text("metadata"),
|
|
searchText: text("search_text"),
|
|
createdAt: numeric("created_at").default(sql`(CURRENT_TIMESTAMP)`),
|
|
updatedAt: numeric("updated_at").default(sql`(CURRENT_TIMESTAMP)`)
|
|
})
|
|
|
|
export const extensionDataFts = sqliteTable("extension_data_fts", {
|
|
dataId: numeric("data_id"),
|
|
searchText: numeric("search_text"),
|
|
extensionDataFts: numeric("extension_data_fts"),
|
|
rank: numeric()
|
|
})
|
|
|
|
export const extensionDataFtsData = sqliteTable("extension_data_fts_data", {
|
|
id: integer().primaryKey(),
|
|
block: blob()
|
|
})
|
|
|
|
export const extensionDataFtsIdx = sqliteTable(
|
|
"extension_data_fts_idx",
|
|
{
|
|
segid: numeric().notNull(),
|
|
term: numeric().notNull(),
|
|
pgno: numeric()
|
|
},
|
|
(table) => [
|
|
primaryKey({ columns: [table.segid, table.term], name: "extension_data_fts_idx_segid_term_pk" })
|
|
]
|
|
)
|
|
|
|
export const extensionDataFtsDocsize = sqliteTable("extension_data_fts_docsize", {
|
|
id: integer().primaryKey(),
|
|
sz: blob()
|
|
})
|
|
|
|
export const extensionDataFtsConfig = sqliteTable("extension_data_fts_config", {
|
|
k: numeric().primaryKey().notNull(),
|
|
v: numeric()
|
|
})
|