mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-07-12 02:41:30 +00:00
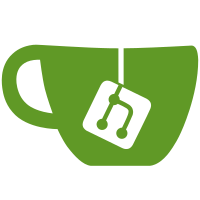
* refactor: add a valibot schema for package registry validation * fix: list view action menu * chore: bump version to 0.1.16 in package.json * refactor: extract supabase package from api * ci: remove NODE_OPTIONS from build step and improve error handling in getLatestNpmPkgVersion function
45 lines
1.3 KiB
TypeScript
45 lines
1.3 KiB
TypeScript
import { findAllArgsInLink } from "@/cmds/quick-links"
|
|
import { Action as ActionSchema, CmdTypeEnum } from "@kksh/api/models"
|
|
import type { AppState } from "@kksh/types"
|
|
import type { CmdValue } from "@kksh/ui/types"
|
|
import { derived, get, writable, type Writable } from "svelte/store"
|
|
|
|
export const defaultAppState: AppState = {
|
|
searchTerm: "",
|
|
highlightedCmd: "",
|
|
loadingBar: false,
|
|
defaultAction: "",
|
|
actionPanel: undefined
|
|
}
|
|
|
|
interface AppStateAPI {
|
|
clearSearchTerm: () => void
|
|
get: () => AppState
|
|
setLoadingBar: (loadingBar: boolean) => void
|
|
setDefaultAction: (defaultAction: string | null) => void
|
|
setActionPanel: (actionPanel?: ActionSchema.ActionPanel) => void
|
|
}
|
|
|
|
function createAppState(): Writable<AppState> & AppStateAPI {
|
|
const store = writable<AppState>(defaultAppState)
|
|
|
|
return {
|
|
...store,
|
|
get: () => get(store),
|
|
clearSearchTerm: () => {
|
|
store.update((state) => ({ ...state, searchTerm: "" }))
|
|
},
|
|
setLoadingBar: (loadingBar: boolean) => {
|
|
store.update((state) => ({ ...state, loadingBar }))
|
|
},
|
|
setDefaultAction: (defaultAction: string | null) => {
|
|
store.update((state) => ({ ...state, defaultAction }))
|
|
},
|
|
setActionPanel: (actionPanel?: ActionSchema.ActionPanel) => {
|
|
store.update((state) => ({ ...state, actionPanel }))
|
|
}
|
|
}
|
|
}
|
|
|
|
export const appState = createAppState()
|