mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-07-12 02:41:30 +00:00
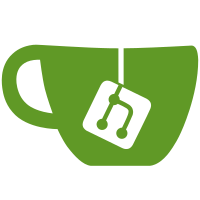
* feat: implement a file streamer for file share Only server with hardcoded file path * bump valibot version * feat: add server-info gRPC module to serve server info * feat: add ssl cert and public key to peers state When peer is online, KK always have know its cert and pub key for future secure communication * feat: add grpc ts package * Enable "/refresh-worker-extension" rest API, grpc version isn't ready yet * update pnpm lock * ci: fix CI by moving protobuf install order * ci: fix * upgrade api due to valibot incompatibility * fix: use fs instead of bun shell to be compatible with windows * skip grpc pkg build on windows * feat: local network file transfer prototype working * fix: grpc build.ts * download next to 14 * ci: add ci env try to fix next * fix: hideRefreshBtn and a few other btns' hide API in iframe ext page * feat: disable NODE_TLS_REJECT_UNAUTHORIZED for extension HMR refresh * fix: manifest json schema with objectWithRest to allow any other fields in package.json * chore: update valibot and related dependencies to version 1.0.0-beta.9 in pnpm-lock.yaml * ci: add protobuf compiler installation to manifest-schema-upload workflow * refactor: move grpc code from jarvis to a separate grpc crate for easier testing * feat(file-transfer): POC multi file + directory file transfer * feat(file-transfer): replace file transfer recursive download in ts with rust * feat(file-transfer): implement on_progress event for file transfer * feat(file-transfer): report progress every 1MB instead of 100 iterations * feat(file-transfer): add progress bar * feat(file-transfer): UI * feat(file-transfer): add file transfer bucket info preview Show total size and number of files * feat(file-transfer): improve UX Show bucket info during confirm; improve progress bar UI, prevent inconsistent width * feat(grpc): skip build in Cloudflare Pages due to missing protoc * refactor: with cargo fix, unused imports removed * ci: debug cloudflare pages env var * fix(grpc): update environment variable access for Cloudflare Pages build check * fix(grpc): add error handling for protoc command in build script * chore: update kkrpc version to 0.0.13, remove kkrpc submodule, and enhance grpc build script logging - Updated kkrpc dependency version from 0.0.12 to 0.0.13 in package.json. - Removed the kkrpc submodule from the project. - Enhanced logging in the grpc build script to include additional Cloudflare Pages environment variables for better debugging. * fix(api): typescript error, remove base.json from tsconfig * chore: update pnpm lock * fix(api): update TypeScript configuration to extend base.json and clean up unused options * refactor(api): update TypeScript configuration to extend path-alias.json and enhance compiler options * fix(api): restore KunkunManifestPermission in PermissionUnion and update valibot import in schema tests * fix: missing trait error * fix: js require replaced with import * test: fix a unit test with a more robust method --------- Co-authored-by: Huakun Shen <huaukun.shen@huakunshen.com>
210 lines
5.7 KiB
Svelte
210 lines
5.7 KiB
Svelte
<script lang="ts">
|
|
import DanceTransition from "@/components/dance/dance-transition.svelte"
|
|
import { appConfig, winExtMap } from "@/stores"
|
|
import { goBackOnEscape } from "@/utils/key"
|
|
import { goHome } from "@/utils/route"
|
|
import { positionToCssStyleString, positionToTailwindClasses } from "@/utils/style"
|
|
import { isInMainWindow } from "@/utils/window"
|
|
import { db } from "@kksh/api/commands"
|
|
import { CustomPosition, ThemeColor, type Position } from "@kksh/api/models"
|
|
import {
|
|
constructJarvisServerAPIWithPermissions,
|
|
// exposeApiToWindow,
|
|
type IApp,
|
|
type IUiIframe
|
|
} from "@kksh/api/ui"
|
|
import { toast, type IUiIframeServer2 } from "@kksh/api/ui/iframe"
|
|
import { Button } from "@kksh/svelte5"
|
|
import { cn } from "@kksh/ui/utils"
|
|
import { getCurrentWindow } from "@tauri-apps/api/window"
|
|
import { goto } from "$app/navigation"
|
|
import { IframeParentIO, RPCChannel } from "kkrpc/browser"
|
|
import { ArrowLeftIcon, MoveIcon, RefreshCcwIcon, XIcon } from "lucide-svelte"
|
|
import { onDestroy, onMount } from "svelte"
|
|
import * as v from "valibot"
|
|
import type { PageData } from "./$types"
|
|
|
|
let { data }: { data: PageData } = $props()
|
|
const { loadedExt, url, extPath, extInfoInDB } = data
|
|
const appWin = getCurrentWindow()
|
|
let iframeRef: HTMLIFrameElement
|
|
let uiControl = $state<{
|
|
iframeLoaded: boolean
|
|
showBackBtn: boolean
|
|
showMoveBtn: boolean
|
|
showRefreshBtn: boolean
|
|
backBtnPosition: Position
|
|
moveBtnPosition: Position
|
|
refreshBtnPosition: Position
|
|
transparentBg: boolean
|
|
}>({
|
|
iframeLoaded: false,
|
|
showBackBtn: true, // if open in new window, hide back button
|
|
showMoveBtn: true,
|
|
showRefreshBtn: true,
|
|
backBtnPosition: "top-left",
|
|
moveBtnPosition: "bottom-left",
|
|
refreshBtnPosition: "top-right",
|
|
transparentBg: false
|
|
})
|
|
|
|
const iframeUiAPI: IUiIframeServer2 = {
|
|
goBack: async () => {
|
|
if (isInMainWindow()) {
|
|
goto("/")
|
|
} else {
|
|
appWin.close()
|
|
}
|
|
},
|
|
hideBackButton: async () => {
|
|
uiControl.showBackBtn = false
|
|
},
|
|
hideMoveButton: async () => {
|
|
uiControl.showMoveBtn = false
|
|
},
|
|
hideRefreshButton: async () => {
|
|
console.log("hideRefreshButton")
|
|
uiControl.showRefreshBtn = false
|
|
},
|
|
showBackButton: async (position?: Position) => {
|
|
console.log("showBackBtn", position)
|
|
|
|
uiControl.showBackBtn = true
|
|
uiControl.backBtnPosition = position ?? "top-left"
|
|
},
|
|
showMoveButton: async (position?: Position) => {
|
|
uiControl.showMoveBtn = true
|
|
uiControl.moveBtnPosition = position ?? "bottom-left"
|
|
},
|
|
showRefreshButton: async (position?: Position) => {
|
|
uiControl.showRefreshBtn = true
|
|
uiControl.refreshBtnPosition = position ?? "top-right"
|
|
},
|
|
getTheme: () => {
|
|
const theme = $appConfig.theme
|
|
return Promise.resolve({
|
|
theme: theme.theme as ThemeColor,
|
|
radius: theme.radius,
|
|
lightMode: theme.lightMode
|
|
})
|
|
},
|
|
async reloadPage() {
|
|
location.reload()
|
|
},
|
|
async setTransparentWindowBackground(transparent: boolean) {
|
|
if (isInMainWindow()) {
|
|
throw new Error("Cannot set background in main window")
|
|
}
|
|
if (transparent) {
|
|
document.body.style.backgroundColor = "transparent"
|
|
} else {
|
|
document.body.style.backgroundColor = ""
|
|
}
|
|
}
|
|
}
|
|
|
|
const serverAPI: Record<string, any> = constructJarvisServerAPIWithPermissions(
|
|
loadedExt.kunkun.permissions,
|
|
loadedExt.extPath
|
|
)
|
|
serverAPI.iframeUi = {
|
|
...serverAPI.iframeUi,
|
|
...iframeUiAPI
|
|
} satisfies IUiIframe
|
|
serverAPI.db = new db.JarvisExtDB(extInfoInDB.extId)
|
|
serverAPI.app = {
|
|
language: () => Promise.resolve("en") // TODO: get locale
|
|
} satisfies IApp
|
|
|
|
function onBackBtnClicked() {
|
|
if (isInMainWindow()) {
|
|
goHome()
|
|
} else {
|
|
appWin.close()
|
|
}
|
|
}
|
|
|
|
function onIframeLoaded() {
|
|
setTimeout(() => {
|
|
iframeRef.focus()
|
|
uiControl.iframeLoaded = true
|
|
}, 300)
|
|
}
|
|
|
|
onMount(() => {
|
|
appWin.show()
|
|
if (iframeRef?.contentWindow) {
|
|
const io = new IframeParentIO(iframeRef.contentWindow)
|
|
const rpc = new RPCChannel(io, { expose: serverAPI })
|
|
// exposeApiToWindow(iframeRef.contentWindow, serverAPI)
|
|
} else {
|
|
toast.warning("iframeRef.contentWindow not available")
|
|
}
|
|
|
|
setTimeout(() => {
|
|
if (!uiControl.iframeLoaded) {
|
|
toast.error("Extension failed to load")
|
|
}
|
|
}, 3_000)
|
|
})
|
|
|
|
onDestroy(() => {
|
|
winExtMap.unregisterExtensionFromWindow(appWin.label)
|
|
})
|
|
</script>
|
|
|
|
<svelte:window on:keydown={goBackOnEscape} />
|
|
{#if uiControl.backBtnPosition && uiControl.showBackBtn}
|
|
<Button
|
|
class={cn("absolute", positionToTailwindClasses(uiControl.backBtnPosition))}
|
|
size="icon"
|
|
variant="outline"
|
|
onclick={onBackBtnClicked}
|
|
style={`${positionToCssStyleString(uiControl.backBtnPosition)}`}
|
|
>
|
|
{#if appWin.label === "main"}
|
|
<ArrowLeftIcon class="w-4" />
|
|
{:else}
|
|
<XIcon class="w-4" />
|
|
{/if}
|
|
</Button>
|
|
{/if}
|
|
{#if uiControl.moveBtnPosition && uiControl.showMoveBtn}
|
|
<Button
|
|
class={cn("absolute", positionToTailwindClasses(uiControl.moveBtnPosition))}
|
|
style={`${positionToCssStyleString(uiControl.moveBtnPosition)}`}
|
|
size="icon"
|
|
variant="outline"
|
|
data-tauri-drag-region
|
|
>
|
|
<MoveIcon data-tauri-drag-region class="w-4" />
|
|
</Button>
|
|
{/if}
|
|
{#if uiControl.refreshBtnPosition && uiControl.showRefreshBtn}
|
|
<Button
|
|
class={cn("absolute", positionToTailwindClasses(uiControl.refreshBtnPosition))}
|
|
style={`${positionToCssStyleString(uiControl.refreshBtnPosition)}`}
|
|
size="icon"
|
|
variant="outline"
|
|
onclick={iframeUiAPI.reloadPage}
|
|
>
|
|
<RefreshCcwIcon class="w-4" />
|
|
</Button>
|
|
{/if}
|
|
|
|
<main class="h-screen">
|
|
<DanceTransition delay={300} autoHide={false} show={!uiControl.iframeLoaded} />
|
|
<iframe
|
|
bind:this={iframeRef}
|
|
class={cn("h-full", {
|
|
hidden: !uiControl.iframeLoaded
|
|
})}
|
|
onload={onIframeLoaded}
|
|
width="100%"
|
|
height="100%"
|
|
frameborder="0"
|
|
src={data.url}
|
|
title={data.extPath}
|
|
></iframe>
|
|
</main>
|