mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-04-20 21:49:16 +00:00
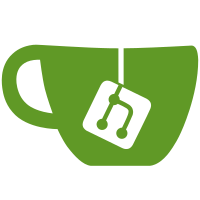
* chore: add vendor submodules * feat: add packages for db,ci,schema,api,jarvis cmds * feat: add tauri-jarvis-plugin * feat: implement extension commands list * fix(desktop): import path errors after packages refactor * chore: add self signed cert * fix: prevent prerender for desktop * fix(desktop): desktop sveltekit static build, use csr for dynamic route * feat: add error handling page and components * refactor: component lib * refactor: move more types, functions and components out of desktop * refactor(ui): more refactor * refactor(ui): move store components to @kksh/ui * ci: add CI for build & test * refactor: rename @kksh/extensions to @kksh/extension * ci: add 2 more ci * ci: fix * fix: CI env var * chore: add changeset * feat: implement extension store item detail view * feat: implement extension store install, uninstall, upgrade * format * revert: upgradable logic, the new one doesn't work yet * refactor: make @kksh/ui dependent only on @kksh/api Reason: @kksh/ui may be published later for building website, all its dependency packages must be also published. To avoid trouble it should be standalone, depend only on packages already published * refactor: cleanup * fixed: some typescript error * chore: got typedoc working on @kksh/api * ci: disable manifest schema upload CI on push
209 lines
6.2 KiB
Rust
209 lines
6.2 KiB
Rust
use super::CommonSystemCmds;
|
|
use std::process::Command;
|
|
|
|
struct Amixer;
|
|
impl Amixer {
|
|
fn cmd_exists() -> bool {
|
|
Command::new("amixer").arg("--version").output().is_ok()
|
|
}
|
|
fn set_volume(value: String) -> anyhow::Result<()> {
|
|
let output = Command::new("amixer")
|
|
.arg("set")
|
|
.arg("Master")
|
|
.arg(value)
|
|
.output()?;
|
|
|
|
if output.status.success() {
|
|
Ok(())
|
|
} else {
|
|
Err(anyhow::anyhow!("Failed to set volume"))
|
|
}
|
|
}
|
|
/// amixer -D pulse set Master 1+ mute
|
|
/// cmd is mute, unmute or toggle
|
|
fn mute_related_cmd(cmd: &str) -> anyhow::Result<()> {
|
|
let output = Command::new("amixer")
|
|
.arg("-D")
|
|
.arg("pulse")
|
|
.arg("set")
|
|
.arg("Master")
|
|
.arg("1+")
|
|
.arg(cmd)
|
|
.output()?;
|
|
|
|
if output.status.success() {
|
|
Ok(())
|
|
} else {
|
|
Err(anyhow::anyhow!("Failed to mute"))
|
|
}
|
|
}
|
|
}
|
|
|
|
struct Pactl;
|
|
impl Pactl {
|
|
fn cmd_exists() -> bool {
|
|
Command::new("pactl").arg("--version").output().is_ok()
|
|
}
|
|
fn set_volume(value: String) -> anyhow::Result<()> {
|
|
let output = Command::new("pactl")
|
|
.arg("set-sink-volume")
|
|
.arg("@DEFAULT_SINK@")
|
|
.arg(value)
|
|
.output()?;
|
|
|
|
if output.status.success() {
|
|
Ok(())
|
|
} else {
|
|
Err(anyhow::anyhow!("Failed to set volume"))
|
|
}
|
|
}
|
|
/// pactl set-sink-mute @DEFAULT_SINK@ true
|
|
/// cmd is true, false or toggle
|
|
fn mute_related_cmd(cmd: &str) -> anyhow::Result<()> {
|
|
let output = Command::new("pactl")
|
|
.arg("set-sink-mute")
|
|
.arg("@DEFAULT_SINK@")
|
|
.arg(cmd)
|
|
.output()?;
|
|
|
|
if output.status.success() {
|
|
Ok(())
|
|
} else {
|
|
Err(anyhow::anyhow!("Failed to mute"))
|
|
}
|
|
}
|
|
}
|
|
|
|
pub struct SystemCmds;
|
|
impl CommonSystemCmds for SystemCmds {
|
|
/// Run nautilus trash://
|
|
fn open_trash() -> anyhow::Result<()> {
|
|
let output = Command::new("nautilus").arg("trash://").output()?;
|
|
match output.status.success() {
|
|
true => Ok(()),
|
|
false => Err(anyhow::anyhow!("Failed to open trash")),
|
|
}
|
|
}
|
|
|
|
fn empty_trash() -> anyhow::Result<()> {
|
|
let output = Command::new("rm")
|
|
.arg("-rf")
|
|
.arg("~/.local/share/Trash/files/*")
|
|
.output()?;
|
|
match output.status.success() {
|
|
true => Ok(()),
|
|
false => Err(anyhow::anyhow!("Failed to empty trash")),
|
|
}
|
|
}
|
|
|
|
fn shutdown() -> anyhow::Result<()> {
|
|
let output = Command::new("shutdown").arg("-h").arg("now").output()?;
|
|
match output.status.success() {
|
|
true => Ok(()),
|
|
false => Err(anyhow::anyhow!("Failed to shutdown")),
|
|
}
|
|
}
|
|
|
|
fn reboot() -> anyhow::Result<()> {
|
|
let output = Command::new("reboot").output()?;
|
|
match output.status.success() {
|
|
true => Ok(()),
|
|
false => Err(anyhow::anyhow!("Failed to reboot")),
|
|
}
|
|
}
|
|
|
|
fn sleep() -> anyhow::Result<()> {
|
|
let output = Command::new("systemctl").arg("suspend").output()?;
|
|
match output.status.success() {
|
|
true => Ok(()),
|
|
false => Err(anyhow::anyhow!("Failed to sleep")),
|
|
}
|
|
}
|
|
|
|
fn set_volume(percentage: u8) -> anyhow::Result<()> {
|
|
if Pactl::cmd_exists() {
|
|
return Pactl::set_volume(format!("{}%", percentage));
|
|
} else if Amixer::cmd_exists() {
|
|
return Amixer::set_volume(format!("{}%", percentage));
|
|
} else {
|
|
return Err(anyhow::anyhow!(
|
|
"No volume control command found (Only Support pactl and amixer)"
|
|
));
|
|
}
|
|
}
|
|
|
|
fn turn_volume_up() -> anyhow::Result<()> {
|
|
if Pactl::cmd_exists() {
|
|
return Pactl::set_volume("+10%".to_string());
|
|
} else if Amixer::cmd_exists() {
|
|
return Amixer::set_volume("10%+".to_string());
|
|
} else {
|
|
return Err(anyhow::anyhow!(
|
|
"No volume control command found (Only Support pactl and amixer)"
|
|
));
|
|
}
|
|
}
|
|
|
|
fn turn_volume_down() -> anyhow::Result<()> {
|
|
if Pactl::cmd_exists() {
|
|
return Pactl::set_volume("-10%".to_string());
|
|
} else if Amixer::cmd_exists() {
|
|
return Amixer::set_volume("10%-".to_string());
|
|
} else {
|
|
return Err(anyhow::anyhow!(
|
|
"No volume control command found (Only Support pactl and amixer)"
|
|
));
|
|
}
|
|
}
|
|
|
|
fn logout_user() -> anyhow::Result<()> {
|
|
let output = Command::new("pkill").arg("Xorg").output()?;
|
|
match output.status.success() {
|
|
true => Ok(()),
|
|
false => Err(anyhow::anyhow!("Failed to logout")),
|
|
}
|
|
}
|
|
|
|
/// amixer -D pulse set Master 1+ toggle
|
|
fn toggle_mute() -> anyhow::Result<()> {
|
|
if Pactl::cmd_exists() {
|
|
Pactl::mute_related_cmd("toggle")
|
|
} else if Amixer::cmd_exists() {
|
|
Amixer::mute_related_cmd("toggle")
|
|
} else {
|
|
Err(anyhow::anyhow!(
|
|
"No volume control command found (Only Support pactl and amixer)"
|
|
))
|
|
}
|
|
}
|
|
/// amixer -D pulse set Master 1+ mute
|
|
fn mute() -> anyhow::Result<()> {
|
|
if Pactl::cmd_exists() {
|
|
Pactl::mute_related_cmd("true")
|
|
} else if Amixer::cmd_exists() {
|
|
Amixer::mute_related_cmd("mute")
|
|
} else {
|
|
Err(anyhow::anyhow!(
|
|
"No volume control command found (Only Support pactl and amixer)"
|
|
))
|
|
}
|
|
}
|
|
|
|
/// amixer -D pulse set Master 1+ unmute
|
|
fn unmute() -> anyhow::Result<()> {
|
|
if Pactl::cmd_exists() {
|
|
Pactl::mute_related_cmd("false")
|
|
} else if Amixer::cmd_exists() {
|
|
Amixer::mute_related_cmd("unmute")
|
|
} else {
|
|
Err(anyhow::anyhow!(
|
|
"No volume control command found (Only Support pactl and amixer)"
|
|
))
|
|
}
|
|
}
|
|
|
|
fn get_selected_files() -> anyhow::Result<Vec<std::path::PathBuf>> {
|
|
Ok(vec![])
|
|
}
|
|
}
|