mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-04-11 17:29:44 +00:00
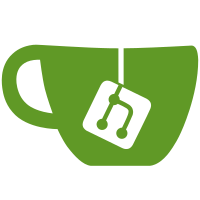
* feat: implement app loader (has performance problem) * feat: enhance command filtering and search functionality - Implement command score filtering for various command types - Add filtered stores for quick links, system commands, and extensions - Update command components to use new filtering mechanism - Improve search experience by dynamically filtering results - Refactor command value handling to use direct name matching
57 lines
1.4 KiB
TypeScript
57 lines
1.4 KiB
TypeScript
import type { Icon } from "@kksh/api/models"
|
|
import { createQuickLinkCommand, getAllQuickLinkCommands } from "@kksh/extension/db"
|
|
import type { QuickLink } from "@kksh/ui/types"
|
|
import { commandScore } from "@kksh/ui/utils"
|
|
import { derived, get, writable, type Writable } from "svelte/store"
|
|
import { appState } from "./appState"
|
|
|
|
export interface QuickLinkAPI {
|
|
get: () => QuickLink[]
|
|
init: () => Promise<void>
|
|
refresh: () => Promise<void>
|
|
createQuickLink: (name: string, link: string, icon: Icon) => Promise<void>
|
|
}
|
|
|
|
function createQuickLinksStore(): Writable<QuickLink[]> & QuickLinkAPI {
|
|
const store = writable<QuickLink[]>([])
|
|
|
|
async function init() {
|
|
refresh()
|
|
}
|
|
|
|
async function refresh() {
|
|
const cmds = await getAllQuickLinkCommands()
|
|
store.set(cmds.map((cmd) => ({ link: cmd.data.link, name: cmd.name, icon: cmd.data.icon })))
|
|
}
|
|
|
|
async function createQuickLink(name: string, link: string, icon: Icon) {
|
|
await createQuickLinkCommand(name, link, icon)
|
|
await refresh()
|
|
}
|
|
|
|
return {
|
|
...store,
|
|
get: () => get(store),
|
|
init,
|
|
refresh,
|
|
createQuickLink
|
|
}
|
|
}
|
|
|
|
export const quickLinks = createQuickLinksStore()
|
|
|
|
// export const quickLinksFiltered = derived([quickLinks, appState], ([$quicklinks, $appState]) => {
|
|
// return $quicklinks.filter((lnk) => {
|
|
// if ($appState.searchTerm.length === 0) {
|
|
// return false
|
|
// }
|
|
// return (
|
|
// commandScore(
|
|
// lnk.name,
|
|
// $appState.searchTerm
|
|
// // []
|
|
// ) > 0.5
|
|
// )
|
|
// })
|
|
// })
|