mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-07-02 22:41:31 +00:00
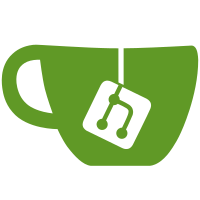
* feat: implement clipboard history preview * feat: clipboard history pagination * refactor: format code * fix: sql schema * feat: add json metadata to unit test * upgrade: js dependencies * upgrade: desktop rust dependencies * fix: clipboard history duplicate key bug when searchTerm clears * upgrade: tauri-plugin-network submodule solve pnpm lock file * fix: grpc package CI * chore: update turbo.json outputs to include dist and build directories * fix: try to fix template-ext-vue tailwind module.export * ci: prevent error when protoc is not installed in CF pages * fix: update writeFile function to accept ReadableStream as data type
32 lines
858 B
TypeScript
32 lines
858 B
TypeScript
import { enum_, type InferOutput } from "valibot"
|
|
|
|
export enum SQLSortOrderEnum {
|
|
Asc = "ASC",
|
|
Desc = "DESC"
|
|
}
|
|
|
|
export const SQLSortOrder = enum_(SQLSortOrderEnum)
|
|
export type SQLSortOrder = InferOutput<typeof SQLSortOrder>
|
|
|
|
export enum SearchModeEnum {
|
|
ExactMatch = "exact_match",
|
|
Like = "like",
|
|
FTS = "fts"
|
|
}
|
|
|
|
export const SearchMode = enum_(SearchModeEnum)
|
|
export type SearchMode = InferOutput<typeof SearchMode>
|
|
|
|
export function convertDateToSqliteString(date: Date) {
|
|
const pad = (num: number) => num.toString().padStart(2, "0")
|
|
|
|
const year = date.getFullYear()
|
|
const month = pad(date.getMonth() + 1) // getMonth() returns 0-11
|
|
const day = pad(date.getDate())
|
|
const hours = pad(date.getHours())
|
|
const minutes = pad(date.getMinutes())
|
|
const seconds = pad(date.getSeconds())
|
|
|
|
return `${year}-${month}-${day} ${hours}:${minutes}:${seconds}`
|
|
}
|