mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-07-15 12:11:33 +00:00
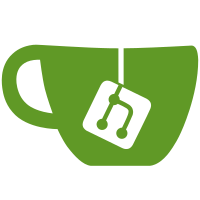
* feat: add ui worker command loading code (not working yet) * feat: add unocss * feat: add-dev-extension page * feat: implemented list view template * feat: implement list view detail view width, add demo extension for dev * fix: resize listview, add metadata component * fix: metadata tag component background color * feat: implement boolean (checkbox), date fields for form template * feat: support default, optional, placeholder for form fields * feat: implemented form view Select Field * feat: markdown view * feat: fixed a markdown schema type error * fix: markdown styling * feat: implement action panel for UI worker template list view * format: format all * chore: bump desktop version * fix: fix search term bind in list view
182 lines
4.7 KiB
TypeScript
182 lines
4.7 KiB
TypeScript
import type { RPCChannel } from "@hk/comlink-stdio/browser"
|
|
import {
|
|
Action,
|
|
app,
|
|
Child,
|
|
expose,
|
|
Form,
|
|
fs,
|
|
Icon,
|
|
IconEnum,
|
|
List,
|
|
Markdown,
|
|
open,
|
|
path,
|
|
security,
|
|
shell,
|
|
toast,
|
|
ui,
|
|
WorkerExtension
|
|
} from "@kksh/api/ui/worker"
|
|
import { IconType } from "@kunkun/api/models"
|
|
|
|
const nums = Array.from({ length: 20 }, (_, i) => i + 1)
|
|
const categories = ["Suggestion", "Advice", "Idea"]
|
|
const itemsTitle = nums.map((n) => categories.map((c) => `${c} ${n}`)).flat()
|
|
const allItems: List.Item[] = itemsTitle.map(
|
|
(title) =>
|
|
new List.Item({
|
|
title,
|
|
value: title,
|
|
defaultAction: "Item Default Action"
|
|
})
|
|
)
|
|
|
|
class ExtensionTemplate extends WorkerExtension {
|
|
async onBeforeGoBack() {
|
|
console.log("onBeforeGoBack")
|
|
// console.log(`Try killing pid: ${this.apiProcess?.pid}`)
|
|
// await this.apiProcess?.kill()
|
|
// console.log("apiProcess killed")
|
|
}
|
|
async onFormSubmit(value: Record<string, any>): Promise<void> {
|
|
console.log("Form submitted", value)
|
|
}
|
|
|
|
async onEnterPressedOnSearchBar(): Promise<void> {
|
|
console.log("Enter pressed on search bar")
|
|
}
|
|
|
|
async load() {
|
|
// console.log("Check screen capture permission:", await security.mac.checkScreenCapturePermission())
|
|
// await security.mac.revealSecurityPane("AllFiles")
|
|
// console.log(await security.mac.verifyFingerprint())
|
|
ui.setSearchBarPlaceholder("Search for items")
|
|
ui.showLoadingBar(true)
|
|
setTimeout(() => {
|
|
ui.showLoadingBar(false)
|
|
}, 2000)
|
|
const { rpcChannel, process } = await shell.createDenoRpcChannel<
|
|
{},
|
|
{
|
|
add(a: number, b: number): Promise<number>
|
|
subtract(a: number, b: number): Promise<number>
|
|
}
|
|
>("$EXTENSION/deno-src/rpc.ts", [], {}, {})
|
|
const api = rpcChannel.getApi()
|
|
await api.add(1, 2).then(console.log)
|
|
await api.subtract(1, 2).then(console.log)
|
|
await process.kill()
|
|
const extPath = await path.extensionDir()
|
|
// console.log("Extension path:", extPath)
|
|
const tagList = new List.ItemDetailMetadataTagList({
|
|
title: "Tag List Title",
|
|
tags: [
|
|
new List.ItemDetailMetadataTagListItem({
|
|
text: "red",
|
|
color: "#ff0000"
|
|
}),
|
|
new List.ItemDetailMetadataTagListItem({
|
|
text: "yellow",
|
|
color: "#ffff00"
|
|
})
|
|
]
|
|
})
|
|
const list = new List.List({
|
|
items: allItems,
|
|
defaultAction: "Top Default Action",
|
|
detail: new List.ItemDetail({
|
|
children: [
|
|
new List.ItemDetailMetadata([
|
|
new List.ItemDetailMetadataLabel({
|
|
title: "Label Title",
|
|
text: "Label Text"
|
|
}),
|
|
new List.ItemDetailMetadataLabel({
|
|
title: "Label Title",
|
|
text: "Label Text",
|
|
icon: new Icon({
|
|
type: IconType.enum.Iconify,
|
|
value: "mingcute:appstore-fill"
|
|
})
|
|
}),
|
|
new List.ItemDetailMetadataSeparator(),
|
|
new List.ItemDetailMetadataLabel({
|
|
title: "Label Title",
|
|
text: "Label Text"
|
|
}),
|
|
new List.ItemDetailMetadataLink({
|
|
title: "Link Title",
|
|
text: "Link Text",
|
|
url: "https://github.com/huakunshen"
|
|
}),
|
|
new List.ItemDetailMetadataLabel({
|
|
title: "Label Title",
|
|
text: "Label Text"
|
|
}),
|
|
tagList
|
|
]),
|
|
new Markdown(`
|
|
# Hello World
|
|
<img src="https://github.com/huakunshen.png" />
|
|
<img src="https://github.com/huakunshen.png" />
|
|
<img src="https://github.com/huakunshen.png" />
|
|
`)
|
|
],
|
|
width: 50
|
|
}),
|
|
actions: new Action.ActionPanel({
|
|
items: [
|
|
new Action.Action({
|
|
title: "Action 1",
|
|
value: "action 1",
|
|
icon: new Icon({ type: IconType.enum.Iconify, value: "material-symbols:add-reaction" })
|
|
}),
|
|
new Action.Action({ title: "Action 2", value: "action 2" }),
|
|
new Action.Action({ title: "Action 3", value: "action 3" }),
|
|
new Action.Action({ title: "Action 4", value: "action 4" })
|
|
]
|
|
})
|
|
})
|
|
|
|
return ui.render(list)
|
|
}
|
|
|
|
async onSearchTermChange(term: string): Promise<void> {
|
|
return ui.render(
|
|
new List.List({
|
|
// items: allItems.filter((item) => item.title.toLowerCase().includes(term.toLowerCase())),
|
|
inherits: ["items", "sections"],
|
|
defaultAction: "Top Default Action",
|
|
detail: new List.ItemDetail({
|
|
children: [
|
|
new List.ItemDetailMetadata([
|
|
new List.ItemDetailMetadataLabel({
|
|
title: "Label Title",
|
|
text: "Label Text"
|
|
})
|
|
])
|
|
// new Markdown(`
|
|
// ## Search results for "${term}"
|
|
// <img src="https://github.com/huakunshen.png" />
|
|
// <img src="https://github.com/huakunshen.png" />
|
|
// <img src="https://github.com/huakunshen.png" />
|
|
// `)
|
|
],
|
|
width: term.length > 3 ? 70 : 30
|
|
})
|
|
})
|
|
)
|
|
}
|
|
|
|
async onListItemSelected(value: string): Promise<void> {
|
|
console.log("Item selected:", value)
|
|
}
|
|
|
|
async onActionSelected(value: string): Promise<void> {
|
|
console.log("Action selected:", value)
|
|
}
|
|
}
|
|
|
|
expose(new ExtensionTemplate())
|