mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-07-03 23:01:30 +00:00
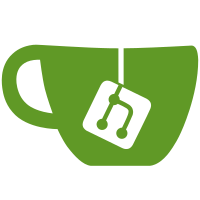
* feat: implement a file streamer for file share Only server with hardcoded file path * bump valibot version * feat: add server-info gRPC module to serve server info * feat: add ssl cert and public key to peers state When peer is online, KK always have know its cert and pub key for future secure communication * feat: add grpc ts package * Enable "/refresh-worker-extension" rest API, grpc version isn't ready yet * update pnpm lock * ci: fix CI by moving protobuf install order * ci: fix * upgrade api due to valibot incompatibility * fix: use fs instead of bun shell to be compatible with windows * skip grpc pkg build on windows * feat: local network file transfer prototype working * fix: grpc build.ts * download next to 14 * ci: add ci env try to fix next * fix: hideRefreshBtn and a few other btns' hide API in iframe ext page * feat: disable NODE_TLS_REJECT_UNAUTHORIZED for extension HMR refresh * fix: manifest json schema with objectWithRest to allow any other fields in package.json * chore: update valibot and related dependencies to version 1.0.0-beta.9 in pnpm-lock.yaml * ci: add protobuf compiler installation to manifest-schema-upload workflow * refactor: move grpc code from jarvis to a separate grpc crate for easier testing * feat(file-transfer): POC multi file + directory file transfer * feat(file-transfer): replace file transfer recursive download in ts with rust * feat(file-transfer): implement on_progress event for file transfer * feat(file-transfer): report progress every 1MB instead of 100 iterations * feat(file-transfer): add progress bar * feat(file-transfer): UI * feat(file-transfer): add file transfer bucket info preview Show total size and number of files * feat(file-transfer): improve UX Show bucket info during confirm; improve progress bar UI, prevent inconsistent width * feat(grpc): skip build in Cloudflare Pages due to missing protoc * refactor: with cargo fix, unused imports removed * ci: debug cloudflare pages env var * fix(grpc): update environment variable access for Cloudflare Pages build check * fix(grpc): add error handling for protoc command in build script * chore: update kkrpc version to 0.0.13, remove kkrpc submodule, and enhance grpc build script logging - Updated kkrpc dependency version from 0.0.12 to 0.0.13 in package.json. - Removed the kkrpc submodule from the project. - Enhanced logging in the grpc build script to include additional Cloudflare Pages environment variables for better debugging. * fix(api): typescript error, remove base.json from tsconfig * chore: update pnpm lock * fix(api): update TypeScript configuration to extend base.json and clean up unused options * refactor(api): update TypeScript configuration to extend path-alias.json and enhance compiler options * fix(api): restore KunkunManifestPermission in PermissionUnion and update valibot import in schema tests * fix: missing trait error * fix: js require replaced with import * test: fix a unit test with a more robust method --------- Co-authored-by: Huakun Shen <huaukun.shen@huakunshen.com>
67 lines
2.6 KiB
Rust
67 lines
2.6 KiB
Rust
use crate::commands::discovery::{Peers, ServiceInfoMod};
|
|
use mdns_sd::ServiceEvent;
|
|
use std::collections::HashMap;
|
|
use sysinfo::System;
|
|
use tauri::{AppHandle, Manager, Runtime};
|
|
use tauri_plugin_network::network::mdns::MdnsService;
|
|
use uuid::Uuid;
|
|
|
|
pub fn setup_mdns(my_port: u16, public_rsa_key: String) -> anyhow::Result<MdnsService> {
|
|
let mdns = MdnsService::new("kunkun")?;
|
|
let id = Uuid::new_v4();
|
|
let mut properties: HashMap<String, String> = HashMap::new();
|
|
if let Some(hostname) = System::host_name() {
|
|
properties.insert("hostname".to_string(), hostname);
|
|
}
|
|
// there seems to be a limit on txt properties, I can't include public key here
|
|
mdns.register(
|
|
&format!("desktop-{}", id),
|
|
&MdnsService::get_default_ips_str(),
|
|
my_port,
|
|
None,
|
|
Some(properties),
|
|
)?;
|
|
Ok(mdns)
|
|
}
|
|
|
|
pub fn handle_mdns_service_evt<R: Runtime>(
|
|
app_handle: &AppHandle<R>,
|
|
rx: mdns_sd::Receiver<ServiceEvent>,
|
|
) {
|
|
let app_handle = app_handle.clone();
|
|
tauri::async_runtime::spawn(async move {
|
|
while let Ok(event) = rx.recv() {
|
|
match event {
|
|
// ServiceEvent::ServiceResolved(info) => {
|
|
// log::info!("Service Resolved: {:?}", info);
|
|
// },
|
|
ServiceEvent::ServiceResolved(info) => {
|
|
log::info!("Service Resolved: {:#?}", info);
|
|
match ServiceInfoMod::from(info).await {
|
|
Ok(service_info) => {
|
|
app_handle.state::<Peers>().add_peer(service_info).await;
|
|
if let Ok(peers) = app_handle.state::<Peers>().peers.lock() {
|
|
log::info!("Peers: {:#?}", peers.clone());
|
|
} else {
|
|
log::error!("Failed to acquire peers lock");
|
|
}
|
|
}
|
|
Err(e) => {
|
|
log::error!("Failed to create ServiceInfoMod: {}", e);
|
|
}
|
|
}
|
|
}
|
|
ServiceEvent::ServiceRemoved(service_type, fullname) => {
|
|
log::info!("Service Removed: {:?} {:?}", service_type, fullname);
|
|
app_handle
|
|
.state::<Peers>()
|
|
.remove_peer(service_type, fullname);
|
|
let peers = app_handle.state::<Peers>().peers.lock().unwrap().clone();
|
|
log::info!("Peers: {:?}", peers);
|
|
}
|
|
_ => {}
|
|
}
|
|
}
|
|
});
|
|
}
|