mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-07-01 22:11:31 +00:00
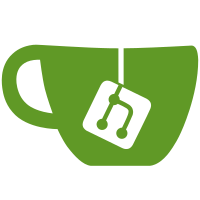
* remove supabase package * upgrade valibot * removed supabase package Migration not complete yet * update submodule * fixed some supabase errors * Add new fields to extension models - Added `id` field to `ExtPublish` - Expanded `DBExtension` with multiple new properties: - `api_version`, `author_id`, `created_at`, - `downloads`, `icon`, `identifier`, - `long_description`, `name`, - `readme`, `short_description`, - and `tarball_size` * Refactor: clean up unused Supabase imports - Removed commented-out Supabase imports from various files to streamline the codebase. - Updated `created_at` type in `ExtPublish` model from `date` to `string` for consistency. * update icon enum to union * fix type errors after removing supabase * format * more types fixed * feat: enhance command handling and update SDK version
96 lines
2.5 KiB
TypeScript
96 lines
2.5 KiB
TypeScript
import { extensions } from "@/stores"
|
|
import { isCompatible } from "@kksh/api"
|
|
import type { ExtPackageJsonExtra } from "@kksh/api/models"
|
|
import { getExtensionsLatestPublishByIdentifier } from "@kksh/sdk"
|
|
import { relaunch } from "@tauri-apps/plugin-process"
|
|
import { check } from "@tauri-apps/plugin-updater"
|
|
import { gt } from "semver"
|
|
import { toast } from "svelte-sonner"
|
|
import { get } from "svelte/store"
|
|
|
|
export async function checkUpdateAndInstall({ beta }: { beta?: boolean } = {}) {
|
|
const update = await check({
|
|
headers: {
|
|
"kk-updater-mode": beta ? "beta" : "stable"
|
|
}
|
|
})
|
|
if (update?.available) {
|
|
const confirmUpdate = await confirm(
|
|
`A new version ${update.version} is available. Do you want to install and relaunch?`
|
|
)
|
|
if (confirmUpdate) {
|
|
await update.downloadAndInstall()
|
|
await relaunch()
|
|
}
|
|
} else {
|
|
toast.info("You are on the latest version")
|
|
}
|
|
}
|
|
|
|
export async function checkSingleExtensionUpdate(
|
|
installedExt: ExtPackageJsonExtra,
|
|
autoupgrade: boolean
|
|
) {
|
|
const {
|
|
data: sbExt,
|
|
error,
|
|
response
|
|
} = await getExtensionsLatestPublishByIdentifier({
|
|
path: {
|
|
identifier: "RAG"
|
|
}
|
|
})
|
|
// const { data: sbExt, error } = await supabaseAPI.getLatestExtPublish(
|
|
// installedExt.kunkun.identifier
|
|
// )
|
|
if (error) {
|
|
return toast.error(
|
|
`Failed to check update for ${installedExt.kunkun.identifier}: ${error} (${response.status})`
|
|
)
|
|
}
|
|
|
|
if (!sbExt) {
|
|
return null
|
|
}
|
|
|
|
if (
|
|
gt(sbExt.version, installedExt.version) &&
|
|
(sbExt.api_version ? isCompatible(sbExt.api_version) : true)
|
|
) {
|
|
if (autoupgrade) {
|
|
await extensions
|
|
.upgradeStoreExtension(sbExt.identifier, sbExt.tarball_path)
|
|
.then(() => {
|
|
toast.success(`${sbExt.name} upgraded`, {
|
|
description: `From ${installedExt.version} to ${sbExt.version}`
|
|
})
|
|
})
|
|
.catch((err) => {
|
|
toast.error(`Failed to upgrade ${sbExt.name}`, { description: err })
|
|
})
|
|
return true
|
|
} else {
|
|
console.log(`new version available ${installedExt.kunkun.identifier} ${sbExt.version}`)
|
|
toast.info(
|
|
`Extension ${installedExt.kunkun.identifier} has a new version ${sbExt.version}, you can upgrade in Store.`,
|
|
{ duration: 10_000 }
|
|
)
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
export async function checkExtensionUpdate(autoupgrade: boolean = false) {
|
|
let upgradedCount = 0
|
|
for (const ext of get(extensions)) {
|
|
const upgraded = await checkSingleExtensionUpdate(ext, autoupgrade)
|
|
if (upgraded) {
|
|
upgradedCount++
|
|
}
|
|
}
|
|
|
|
if (upgradedCount > 0) {
|
|
toast.info(`${upgradedCount} extensions have been upgraded`)
|
|
}
|
|
}
|