mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-07-11 18:31:30 +00:00
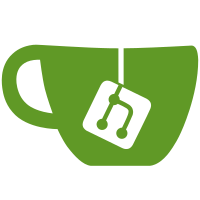
* Add some experiment code for crypto crate * feat: add crypto crate with ssl, rsa, ed25519 for https, encryption, signing * Implement aes encryption helper in crypto crate * ci: add dep for CI rust test (protobuf) * fix: try to fix window CI with next * fix: CI * ci: add dep for ubuntu * ci: fix * fix: openssl lib path in CI * fix: CI * update applications-rs, disable some tests * fix: CI * feat: add file transfer grpc proto and server setup * CI: try to fix CI * fix: missing proto in build.rs * ci: add cargo build before cargo test * fix: grpc file descriptor * ci: fix CI by removing a redundant main.rs * fix: disable local windows test in applications-rs which fails CI * ci: run CI rust test only on ubuntu, windows is failing. will be handled in another PR * fix: vue template * fix: allow unused variable * fix: remove node:buffer type from api shell.ts to avoid frontend build error * try to fix test in create-kunkun * upgrade api to 0.0.44, remove node:buffer * upgrade next template to 15 * feat: turn the default server into a https server * feat: make SSL certificate loadable from env * feat: add conditional SSL cert in debug mode, use local default cert, in production generate new self-signed cert every time app starts * chore: add vscode debug config * feat: add server public key * feat: setup sqlite db encryption * fix: settings hotkey * chore: add .gitkeep * ci: add node-fetch to dep for api package
55 lines
1.4 KiB
Rust
55 lines
1.4 KiB
Rust
use aes::cipher::{BlockDecryptMut, BlockEncryptMut, KeyIvInit};
|
|
use block_padding::Pkcs7;
|
|
use cbc::Decryptor as CbcDec;
|
|
use cbc::Encryptor as CbcEnc;
|
|
type Aes256CbcEnc = CbcEnc<aes::Aes256>;
|
|
type Aes256CbcDec = CbcDec<aes::Aes256>;
|
|
|
|
pub struct Aes256Cbc {
|
|
encryptor: Aes256CbcEnc,
|
|
decryptor: Aes256CbcDec,
|
|
}
|
|
|
|
impl Aes256Cbc {
|
|
pub fn new(key: [u8; 32], iv: [u8; 16]) -> Self {
|
|
Self {
|
|
encryptor: Aes256CbcEnc::new(&key.into(), &iv.into()),
|
|
decryptor: Aes256CbcDec::new(&key.into(), &iv.into()),
|
|
}
|
|
}
|
|
|
|
pub fn encrypt(&self, data: &[u8]) -> Vec<u8> {
|
|
self.encryptor.clone().encrypt_padded_vec_mut::<Pkcs7>(data)
|
|
}
|
|
|
|
pub fn decrypt(&self, data: &[u8]) -> Vec<u8> {
|
|
// let mut buf = data.to_vec();
|
|
self.decryptor
|
|
.clone()
|
|
.decrypt_padded_vec_mut::<Pkcs7>(data)
|
|
.unwrap()
|
|
}
|
|
}
|
|
|
|
#[cfg(test)]
|
|
mod tests {
|
|
use super::*;
|
|
|
|
#[test]
|
|
fn test_encrypt_decrypt() {
|
|
let aes = Aes256Cbc::new([0; 32], [0; 16]);
|
|
let encrypted = aes.encrypt(b"kunkun");
|
|
let decrypted = aes.decrypt(&encrypted);
|
|
assert_eq!(decrypted, b"kunkun");
|
|
}
|
|
|
|
#[test]
|
|
fn test_encrypt_huge_chunk_data() {
|
|
let aes = Aes256Cbc::new([0; 32], [0; 16]);
|
|
let data = vec![0; 1024 * 1024];
|
|
let encrypted = aes.encrypt(&data);
|
|
let decrypted = aes.decrypt(&encrypted);
|
|
assert_eq!(decrypted, data);
|
|
}
|
|
}
|