mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-04-04 14:46:42 +00:00
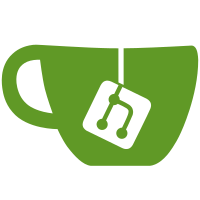
* feat: implement clipboard history preview * feat: clipboard history pagination * refactor: format code * fix: sql schema * feat: add json metadata to unit test * upgrade: js dependencies * upgrade: desktop rust dependencies * fix: clipboard history duplicate key bug when searchTerm clears * upgrade: tauri-plugin-network submodule solve pnpm lock file * fix: grpc package CI * chore: update turbo.json outputs to include dist and build directories * fix: try to fix template-ext-vue tailwind module.export * ci: prevent error when protoc is not installed in CF pages * fix: update writeFile function to accept ReadableStream as data type
40 lines
1.4 KiB
TypeScript
40 lines
1.4 KiB
TypeScript
import fs from "fs"
|
|
import path from "path"
|
|
import tls from "tls"
|
|
import { fileURLToPath } from "url"
|
|
import grpc from "@grpc/grpc-js"
|
|
import * as kk from "./src/protos/kunkun"
|
|
|
|
// Create channel credentials with SSL verification disabled
|
|
const filepath = fileURLToPath(import.meta.url)
|
|
const __dirname = path.dirname(filepath)
|
|
|
|
const certPath = path.join(__dirname, "../tauri-plugins/jarvis/self_signed_certs/cert.pem")
|
|
const credentials = grpc.credentials.createSsl(fs.readFileSync(certPath))
|
|
|
|
// const client = new kk.kunkun.KunkunClient("localhost:9559", credentials) // trust SSL cert
|
|
|
|
// const originalCreateSecureContext = tls.createSecureContext
|
|
// tls.createSecureContext = (options) => {
|
|
// if (options && options.rejectUnauthorized === false) {
|
|
// options.checkServerIdentity = () => undefined // Skip validation
|
|
// }
|
|
// return originalCreateSecureContext(options)
|
|
// }
|
|
|
|
const insecureSslCredentials = grpc.credentials.createSsl(
|
|
null, // Pass `null` to skip validation
|
|
null, // Client key (not used here)
|
|
null // Client certificate (not used here)
|
|
)
|
|
const client = new kk.kunkun.KunkunClient("localhost:9559", insecureSslCredentials) // skip SSL cert validation
|
|
client.ServerInfo(new kk.kunkun.Empty(), (err, response) => {
|
|
console.log(response)
|
|
console.log("public_key", response?.public_key)
|
|
console.log("ssl_cert", response?.ssl_cert)
|
|
if (err) {
|
|
console.error(err)
|
|
}
|
|
})
|
|
// To trust any SSL cert, set NODE_TLS_REJECT_UNAUTHORIZED='0'
|