mirror of
https://github.com/kunkunsh/kunkun.git
synced 2025-07-05 23:51:32 +00:00
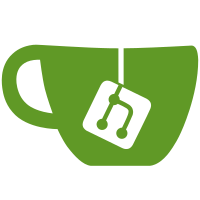
* feat: implement clipboard history preview * feat: clipboard history pagination * refactor: format code * fix: sql schema * feat: add json metadata to unit test * upgrade: js dependencies * upgrade: desktop rust dependencies * fix: clipboard history duplicate key bug when searchTerm clears * upgrade: tauri-plugin-network submodule solve pnpm lock file * fix: grpc package CI * chore: update turbo.json outputs to include dist and build directories * fix: try to fix template-ext-vue tailwind module.export * ci: prevent error when protoc is not installed in CF pages * fix: update writeFile function to accept ReadableStream as data type
46 lines
1.4 KiB
TypeScript
46 lines
1.4 KiB
TypeScript
import fs from "fs"
|
|
import os from "os"
|
|
import path from "path"
|
|
import { fileURLToPath } from "url"
|
|
import { $ } from "bun"
|
|
|
|
// skip this if on Windows, protoc-gen-ts is not supported
|
|
if (os.platform() === "win32") {
|
|
console.log("Skipping build on Windows")
|
|
process.exit(0)
|
|
}
|
|
console.log("process.env.CF_PAGES", Bun.env.CF_PAGES)
|
|
console.log("process.env.CF_PAGES_URL", Bun.env.CF_PAGES_URL)
|
|
console.log("process.env.CF_PAGES_BRANCH", Bun.env.CF_PAGES_BRANCH)
|
|
console.log("process.env.CF_PAGES_COMMIT_SHA", Bun.env.CF_PAGES_COMMIT_SHA)
|
|
if (Bun.env.CF_PAGES_URL) {
|
|
console.warn("Skipping build in Cloudflare Pages, as cloudflare pages does not have protoc")
|
|
process.exit(0)
|
|
}
|
|
|
|
const filepath = fileURLToPath(import.meta.url)
|
|
const __dirname = path.dirname(filepath)
|
|
|
|
const srcPath = path.join(__dirname, "src")
|
|
if (!fs.existsSync(srcPath)) {
|
|
fs.mkdirSync(srcPath)
|
|
}
|
|
|
|
fs.rmSync(path.join(__dirname, "src/protos"), { recursive: true, force: true })
|
|
const protosDir = path.join(__dirname, "protos")
|
|
// find path to protoc command
|
|
const protocPath = Bun.which("protoc")
|
|
if (!protocPath) {
|
|
console.warn("protoc not found")
|
|
process.exit(0)
|
|
}
|
|
for (const file of fs.readdirSync(protosDir)) {
|
|
if (file.endsWith(".proto")) {
|
|
try {
|
|
await $`${protocPath} --plugin=protoc-gen-ts=./node_modules/.bin/protoc-gen-ts --ts_out=./src -I . ./protos/${file}`
|
|
} catch (error) {
|
|
console.error(error)
|
|
}
|
|
}
|
|
}
|